zillow
Question 1)
Given a string, write a routine that converts the string to a long, without using the
built in functions that would do this. Describe what (if any) limitations the code has. For
example:
long stringToLong(String s)
{
/* code goes here to convert a string to a long */
}
void test()
{
long i = stringToLong("123");
if (i == 123)
// success
else
// failure
}
/*
* Complete the function below.
*/
static long parseLong(String str) throws Exception {
if (str == null || str.length() == 0) {
throw new Exception("Invalid number.");
}
long result = 0;
int len = str.length();
boolean isPositive = true;
int start = 0; // first number
// check sign
if (str.charAt(start) == '+') {
isPositive = true;
start++;
} else if (str.charAt(start) == '-') {
isPositive = false;
start++;
}
// check if valid
if (start == len || (str.charAt(start) == '0' && start != len - 1)) {
throw new Exception("Invalid number.");
}
for (int i = start; i < len; i++) {
char c = str.charAt(i);
if (c >= '0' && c <= '9') {
if (result > Long.MAX_VALUE / 10) {
throw new Exception("Number overflow.");
}
if (result == Long.MAX_VALUE / 10 && i == len - 1) {
if (isPositive && c > '7') {
throw new Exception("Number overflow.");
} else if (!isPositive && c > '8') {
throw new Exception("Number Overflow.");
} else if (!isPositive && c == '8') {
return Long.MIN_VALUE;
}
}
result = result * 10;
result += (long) (c - '0');
} else {
throw new Exception("Invalid number.");
}
}
return result;
}
Question 2) Implement insert and delete in a tri-nary tree. A tri-nary tree is much like a binary
tree but with three child nodes for each parent instead of two -- with the left node being values
less than the parent, the right node values greater than the parent, and the middle nodes values
equal to the parent.
For example, suppose I added the following nodes to the tree in this order: 5, 4, 9, 5, 7, 2, 2.
The resulting tree would look like this:
5
/ | \
4 5 9
/ /
2 7
|
2
/* PLEASE DO NOT UNCOMMENT THIS BLOCK
import java.io.IOException;
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.util.*;
// No other imports are permitted
// The following definitions of Tree and Node are provided.
// insert and delete will be methods of class Tree.
public class Tree {
private class Node {
private int val;
private Node left = null;
private Node right = null;
private Node mid = null;
public Node(int val) {
this.val = val;
}
}
private Node root;
*/
/*
* Please complete this method.
* Inserts val into the tree.
*/
public void insert(int val) {
if (root == null) {
root = new Node(val);
return;
}
insert(root, val);
}
private void insert(Node root, int val) {
if (root == null) {
return;
} else if (root.val == val) {
Node curr = root;
while (curr.mid != null) {
curr = curr.mid;
}
curr.mid = new Node(val);
} else if (root.val > val) {
if (root.left != null) {
insert(root.left, val);
} else {
root.left = new Node(val);
}
} else if (root.val < val) {
if (root.right != null) {
insert(root.right, val);
} else {
root.right = new Node(val);
}
}
}
/*
* Please complete this method.
* Deletes only one instance of val from the tree.
* If val does not exist in the tree, do nothing.
*/
public void delete(int val) {
root = delete(root, val);
}
private Node delete(Node root, int val) {
if (root == null) {
return root;
}
if (root.val == val) {
// delete mid
if (root.mid != null) {
root.mid = delete(root.mid, val);
} else if (root.right != null) {
// get most left-bottom one from right subtree
Node curr = root.right;
while (curr.left != null) {
curr = curr.left;
}. from: 1point3acres.com/bbs
int smallest = curr.val;
root.val = smallest;
root.right = delete(root.right, smallest);
} else { // replace root with it's left
root = root.left;
}
} else if (root.val > val) {
root.left = delete(root.left, val);
} else if (root.val < val) {
root.right = delete(root.right, val);
}
return root;
}
Question 3) Nth fib
Question 4)css
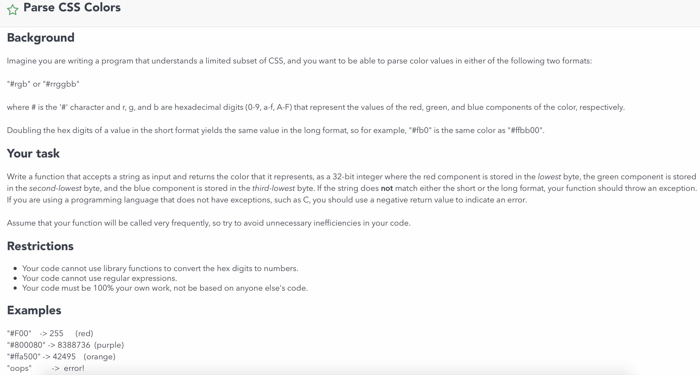